HTML¶
Download Demo¶
To download the demo code for this lecture, run the following:
$ hbget html-combo --demo
Intro¶
What is HTML?¶
HTML stands for HyperText Markup Language
Markup languages are used to categorize content
What is HTML? HTML stands for HyperText Markup Language. Markup
languages are used to organize text into logical sections.
<h1>What is HTML?</h1>
<p>
HTML stands for <cite>HyperText Markup Language</cite>.
</p>
<p>
Markup languages are used to organize text into logical
sections.
</p>
Goals¶
Components of HTML syntax
Enough HTML to make you dangerous
How to build forms in HTML
HTML Syntax¶
Elements¶
A fundamental unit of HTML is called an element
Some elements contain text
<p>HTML is cool.</p>
Start the element with an open tag (
<p>
)End with a close tag (
</p>
)
Some elements don’t contain text content
<img src="/static/cat.png" alt="cat looking out a window">
They comprise of a single, self-closing tag
Attributes¶
Attributes are used to configure elements
<input type="password">
If you don’t explicitly add attributes, you’ll use the default values
To override defaults, add
attr="value"
to the element’s tag
Boolean attributes default to false
You can explicitly set their value to true
A required input¶<input required="true">
But most prefer using this shortcut
<input required>
Entities¶
Entities are shortcuts for displaying special characters
They’re also used to display characters that are reserved for HTML syntax
Character |
Entity |
---|---|
& |
|
< |
|
> |
|
“ |
|
More entities
You can view an official list of all available entities here.
Comments¶
Comments are not displayed by the browser, but still viewable in source
<!-- This is a comment -->
<!--
Everything in between is ignored, so
your comment can contain multiple lines
-->
Whitespace¶
Whitespace is almost insignificant
HTML squeezes any amount of whitespace into a single space character
<p>
This is one line.
This is another line.
</p>
This is one line. This is another line.
You can add newline characters with the line-break tag (<br>
)
<p>
This is one line.<br>
This is another line.
</p>
This is one line.
This is another line.
Using <br> is not a good practice, but fine while learning
In reality, you wouldn’t use <br>
to add a visual line-break. Instead, you
would use CSS to adjust the amount of space between two lines.
HTML Basics¶
The Most Minimal Webpage¶
<!doctype html>
<html>
<head>
<!-- Metadata goes here -->
</head>
<body>
<!-- Actual contents of page goes here -->
</body>
</html>
doctype declaration tells the browser that the page is written in HTML
All HTML should be contained in the html element
head is for metadata content
body should contain content that the user should see
The most basic webpage
The example above is a minimal HTML skeleton. It is not the standard, basic skeleton. A standard HTML page for the web looks like this:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Title Goes Here</title>
</head>
<body>
<!-- Page content goes here -->
</body>
</html>
Browser Dev Tools¶
Firefox, Chrome, and Safari have special developer tools used for debugging HTML, CSS, and JavaScript on the web
You may need to google how to enable developer tools for your browser of choice
To open the HTML Inspector tool:
Right click on page → Inspect element
The Inspector allows you to:
View the HTML source for a page
Temporarily edit HTML
View style properties that have been applied to an HTML element
And more!
ID vs. Class¶
All elements can have attributes called id and class
The id attribute is used to uniquely identify an element
It’s up to you to make sure each id is unique!
<p id="intro">
Welcome!
</p>
<p id="about">
This is my homepage.
</p>
The class attribute is used to categorize elements
Multiple elements can belong to the same class
<a class="nav-link">
Home
</a>
<a class="nav-link">
About
</a>
Block Elements vs. Inline Elements¶
All elements have a default display type
Two basic display types are block and inline
More display types
Block and inline aren’t the only display types. Other display types include:
flex
grid
list-item
Block Elements¶
Start on a new line
Take up the whole width of their containing element
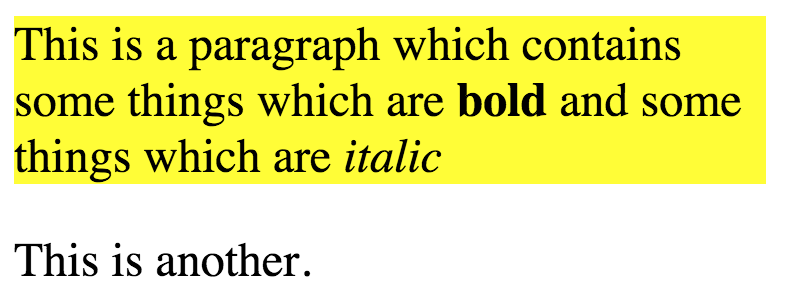
Examples of block elements:
Paragraph (
<p>
)Headings (
<h1>
,<h2>
,<h3>
, etc.)Table (
<table>
)Unordered list (
<ul>
), ordered list (<ol>
)List item (
<li>
)
<h1>Block Elements</h1>
<p>
All of the elements below are block elements.
</p>
<h2>Lists</h2>
<ul>
<li>First item</li>
<li>Second item</li>
</ul>
<ol>
<li>First item</li>
<li>Second item</li>
</ol>
<h2>Table</h2>
<table>
<tr>
<td>print</td>
<td>Print something to the screen</td>
</tr>
<tr>
<td>list</td>
<td>The list constructor function</td>
</tr>
</table>
Inline Elements¶
Sit on a line together
Don’t break the flow of your document
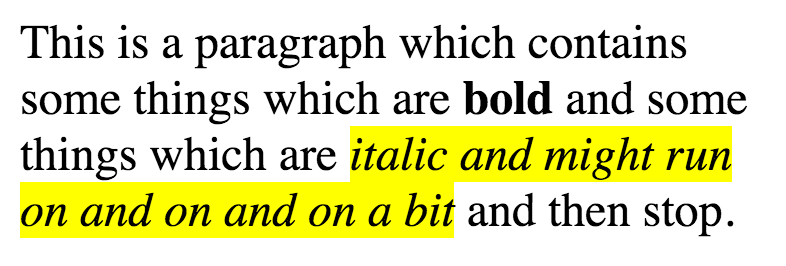
Examples of inline elements:
Hyperlink (
<a>
)Image (
<img>
)Strong (
<strong>
)Emphasis (
<em>
)
<img src="cat2.gif" height="70" alt="cat playing the drums">
<h1>
Contact Us!
</h1>
<p>
Find us on <a href="https://facebook.com">Facebook</a>.
Seriously, <strong>please contact us on Facebook</strong>,
otherwise we <em>might</em> not recieve your message.
</p>
div and span¶
When your content isn’t a paragraph or table or hyperlink (…etc.),
you can use div or span
div is a generic block element
span is a generic inline element
Use id and/or class to label what the element is supposed to be
<div
id="order-confirmation"
class="confirmation-window"
>
<div class="window-body">
<p>
Your order has been placed! to change your order,
please call us at <span class="phone">(555) 555-MELON</span>.
</p>
</div>
<div class="window-footer">
<button>
Close window
</button>
</div>
</div>
Would We Use Block or Inline for…¶
|
|
How to Write Forms¶
The form Element¶
Forms accept user input and send it to a server for processing
<form>
<input type="text" name="username">
<input type="password" name="password">
<input type="submit">
</form>
Form Input Elements¶
Forms can have multiple fields that are defined with form input elements
They’re used to set the name of a field
Sometimes they’re used to define possible values a user can select
There are different types of form input elements:
The input element (
<input>
) comes in different typesText
Checkbox
Radio (radio button)
The select element (
<select>
) is a dropdown menutextarea (
<textarea>
) is for inputting text that is longer than a phrase
A Basic Form Input¶
<input
type="text"
name="username"
value="ball00ni"
>
type is used to configure the kind of input you want
name is used to set the name of a field
value is optional — it is usually set by the user
If a value is defined, it becomes the default value for the field
Label Element: Accessibility¶
The label element allows screen readers to read out the label when users are focused on the element.
The label element also increases the click area - if a user clicks the text inside the label element, it will act as if the user clicked the input element itself.
In order for this functionality to work properly, the for attribute of the label element must be the same as the id of the related input element.
<form>
<label for="user-email">Email</label>
<input type="text" name="email" id="user-email">
</form>
Text Input¶
<form>
<label for="user-email">Email</label>
<input type="text" name="email" id="user-email">
</form>
Radio Buttons¶
Radio buttons are used when the user should select just one option
Each group of radio buttons should have the same name
<form>
Choose a shipping method:
<input type="radio" name="shipping" value="standard" id="standard-shipping">
<label for="standard-shipping">Standard shipping (5-7 days)</label>
<input type="radio" name="shipping" value="expedited" id="expedited-shipping">
<label for="expedited-shipping">Expedited shipping (1-3 days)</label>
</form>
Checkboxes¶
Checkboxes are used when the user can select many options
Each group of checkboxes should have the same name
<form>
What music genres do you like?
<input type="checkbox" name="genres" value="pop" id="pop-genre">
<label for="pop-genre">Pop</label>
<input type="checkbox" name="genres" value="hiphop" id="hiphop-genre">
<label for="hiphop-genre">Hip Hop</label>
<input type="checkbox" name="genres" value="other" id="other-genre">
<label for="other-genre">Other</label>
</form>
Textareas¶
<form>
<label for="about-me">Tell us about yourself:</label>
<textarea name="about-me" id="about-me"></textarea>
</form>
Submit Button¶
There are two ways to create a “submit” button:
<form>
<input type="submit">
</form>
<form>
<button type="submit">Log In</button>
</form>
Code Style¶
Quotation Marks¶
Double-quotes are standard (but HTML doesn’t care either way)
<!-- Avoid doing this! -->
<input type='text' name='username'>
<!-- Do this instead -->
<input type="text" name="username">
Line Length¶
Keep your line-length to < 80 characters by adding line-breaks
<!-- Avoid doing this! -->
<p>This paragraph is pretty long, so it will go over the character limit and make things really hard to read</p>
<!-- Do this instead -->
<p>
This paragraph is pretty long, so it will go over the character
limit and make things really hard to read.
</p>
You can also add line-breaks inside a tag
<!-- Avoid doing this! -->
<img id="wow" src="/static/very/long/url/here.png" alt="A dog looking into the sunset">
<!-- Do this instead -->
<img
id="wow"
src="/static/very/long/url/here.png"
alt="A dog looking into the sunset"
>
Indentation¶
Use indentation to show that content belongs inside an element
<!-- Avoid doing this! -->
<div class="article"><div class="header"><h1>Title of an Article</h1></div>
<div class="intro"><p>Hi</p></div></div>
<!-- Do this instead -->
<div class="article">
<div class="header">
<h1>Title of an Article</h1>
</div>
<div class="intro">
<p>Hi</p>
</div>
</div>
Accessibility Considerations¶
Semantic HTML¶
Writing semantic HTML means using the most appropriate and specific HTML element possible, according to its intended purpose
HTML elements already have built in accessibility functionality, so choosing the best element for its intended purpose creates an optimized experience
General Tips for Semantic HTML¶
Don’t use a
div
if there is a more appropriate HTML element you can use<!-- Avoid doing this! --> <div>Play video</div> <!-- Do this instead --> <button>Play video</button>
Use header tags (
h1
,h2
, etc ) for organization rather than styling. Screen readers use these tags to navigate pages.There should only be one
h1
element per page. Think ofh1
like the title of a book,h2
as chapter names, and so on and so forth.
The alt Attribute¶
alt: the value for this attribute is what is read aloud by a screen reader; if it is not provided, the screen reader will read out the value of the src attribute
<!-- Avoid doing this! -->
<img
id="cat"
src="https://www.publicdomainpictures.net/pictures/320000/nahled/silver-tabby-cat-looking-out-window.jpg"
>
<!-- Do this instead -->
<img
id="cat"
src="https://www.publicdomainpictures.net/pictures/320000/nahled/silver-tabby-cat-looking-out-window.jpg"
alt="cat leaning over chair looking out of window"
>
Best Practices for alt tags
Here are some questions to ask yourself when writing alt text:
Is it redundant? In other words, will the user experience be unaffected if it is not read aloud by a screen reader? If so, the value of the alt attribute can be an empty string
""
Example: stock photos
Does it have a function? If so, the value for the alt attribute should be meaningful.
Example: If the image is a link and an icon, the value should be where the link goes, rather than a description of the icon
What is the context? In other words, what information about this image is important?
Example: An image of food on a recipe site may emphasize instruction-related details while an image of food on a food blog may emphasize the decadence of the image
You can find examples of good and bad alt tags here
The lang Attribute¶
Adding the lang attribute ensures screen readers read content in the correct language and dialect
<html lang="en"> <!-- All the HTML --> </html>
The lang attribute can also be applied to child HTML elements
Values for the lang attribute can include dialects: ex. en-US, en-GB, es-ES, es-MX
Looking Ahead¶
Coming Up¶
CSS
How the web works
Cookies & sessions
JavaScript
Bootstrap
Resources¶
- MDN HTML Documentation: HTML elements reference
A list of all HTML elements — click on a particular element to see examples
- MDN HTML Documentation: The Input (Form input) element
A list of different types of input elements — click on a type to see examples
Further Study¶
- MDN Introduction to HTML: Document and website structure
How to write semantic HTML
- MDN Learn web development: Accessibility
How to ensure your website is accessible to all
- Google Developers: Web Fundamentals
Great resource to study after Hackbright